JavaScript Examples Part 3 for solving requirements with the Frontend Web Assets
Note: These features are part of the Visforms Subscription and are not included in the free Visforms version.
Age verification and conditional fields
Topics
The following topics are included in the example:
- Only run the code after the HTML document has been initialized.
- Only run the code after the form has been initialized.
- React to changes made by the user.
- Determine the date value as text from a date field.
- Calculate with a date value as text and a date object.
- Calculate the age in years taking leap years with leap days into account.
- Address affected fields based on their field ID.
- Make a listbox field invisible.
- Set options for a listbox field.
- Deselect the current option for a listbox field.
Description
The user selects a date of birth. Depending on whether the resulting age means adulthood or not, different additional fields are displayed. If no date is selected, no additional fields are visible. The additional fields for adulthood and minority are all conditional fields.
The display of these conditional fields is controlled by the selection in a control field of the list box type that is later invisible:
- If no selection is made, no additional fields are displayed.
- If option 0 is selected with value 0, the fields for minority are displayed.
- If option 1 is selected with value 1, the fields for adulthood are displayed.
The selection of the invisible control field of the list box type is carried out by the JavaScript code. The JavaScript code calculates the age based on the selected date of birth and sets the corresponding option of the control field. If no date of birth is set or if it is reset by the user, the control list box is set to no selection.
Any number of conditional fields can be displayed and hidden with this logic (age of majority or minor or no information).
The form fields
The form has the following 4 fields:
- birth-date: Field of type Date: Selection of the date of birth.
- listbox-age’: Field of type Listbox:
Listbox as control field with the 3 states:- Nothing selected.
- 0 selected.
- 1 selected.
- text-minor: Field of type Text:
Text input for the case of ‘minor’. Conditional field, only appears for listbox = 0. - text-adult: Field of type Text:
Text input for the case ‘adult’. Conditional field, only appears for listbox = 1.
The form
The form without its own JavaScript code
The control field is still visible. The options in the control field are still selected manually. The JavaScript code later automatically selects the options based on the selected date of birth.
No value selected in the control field.
Neither the text-minor field nor the text-adult field are visible.
Value 0 selected in the control field.
The field text-minor is visible.
Value 1 selected in the control field.
The field text-adult is visible.
The form with its own JavaScript code
The control field is hidden by the JavaScript code. The JavaScript code automatically selects the options in the control field based on the selected birth date.
No birth date selected.
Neither the text-minor field nor the text-adult field are visible.
A young birth date selected.
The text-minor field is visible.
An older birth date selected.
The text-adult field is visible.
The form configuration
The JavaScript code is entered in the form configuration, tab Frontend Webassets on the sub-tab Form in the options field JavaScript.
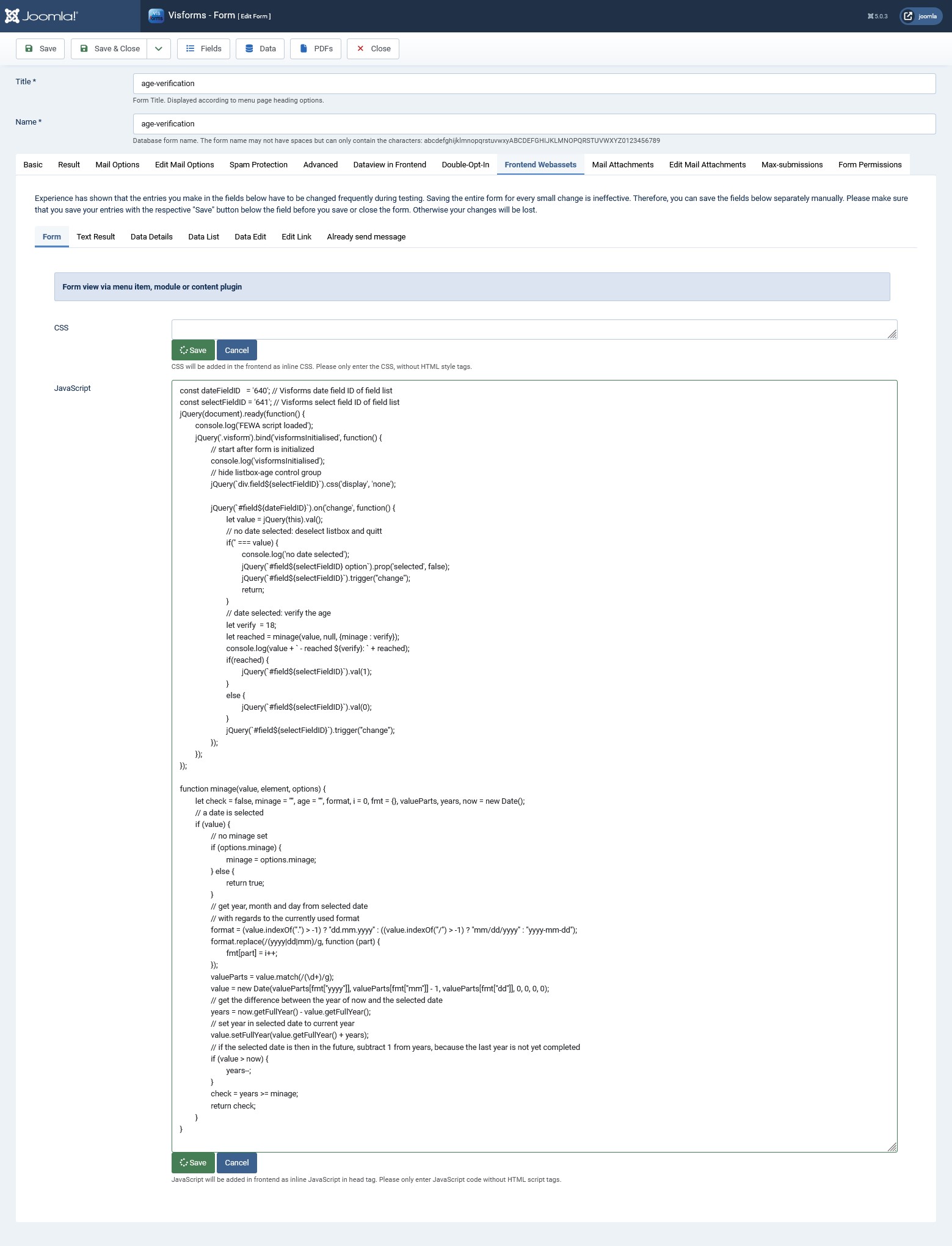
The JavaScript code
const dateFieldID = '640'; // Visforms date field ID of field list
const selectFieldID = '641'; // Visforms select field ID of field list
jQuery(document).ready(function() {
console.log('FEWA script loaded');
jQuery('.visform').bind('visformsInitialised', function() {
// start after form is initialized
console.log('visformsInitialised');
// hide listbox-age control group
jQuery(`div.field${selectFieldID}`).css('display', 'none');
jQuery(`#field${dateFieldID}`).on('change', function() {
let value = jQuery(this).val();
// no date selected: deselect listbox and quitt
if('' === value) {
console.log('no date selected');
jQuery(`#field${selectFieldID} option`).prop('selected', false);
jQuery(`#field${selectFieldID}`).trigger("change");
return;
}
// date selected: verify the age
let verify = 18;
let reached = minage(value, null, {minage : verify});
console.log(value + ` - reached ${verify}: ` + reached);
if(reached) {
jQuery(`#field${selectFieldID}`).val(1);
}
else {
jQuery(`#field${selectFieldID}`).val(0);
}
jQuery(`#field${selectFieldID}`).trigger("change");
});
});
});
function minage(value, element, options) {
let check = false, minage = "", age = "", format, i = 0, fmt = {}, valueParts, years, now = new Date();
// a date is selected
if (value) {
// no minage set
if (options.minage) {
minage = options.minage;
} else {
return true;
}
// get year, month and day from selected date
// with regards to the currently used format
format = (value.indexOf(".") > -1) ? "dd.mm.yyyy" : ((value.indexOf("/") > -1) ? "mm/dd/yyyy" : "yyyy-mm-dd");
format.replace(/(yyyy|dd|mm)/g, function (part) {
fmt[part] = i++;
});
valueParts = value.match(/(\d+)/g);
value = new Date(valueParts[fmt["yyyy"]], valueParts[fmt["mm"]] - 1, valueParts[fmt["dd"]], 0, 0, 0, 0);
// get the difference between the year of now and the selected date
years = now.getFullYear() - value.getFullYear();
// set year in selected date to current year
value.setFullYear(value.getFullYear() + years);
// if the selected date is then in the future, subtract 1 from years, because the last year is not yet completed
if (value > now) {
years--;
}
check = years >= minage;
return check;
}
}
The following adjustments to your situation are necessary:
- const dateFieldID = ‘640' The Visforms date field ID from the field list.
- const selectFieldID = ‘641' The Visforms listbox field ID from the field list (control field).
- let verify = 18 The age to be checked.
In the case of adulthood or minority, no change is necessary.
The function ‘minage()’ is a direct copy from the Visforms code for validation. This function is included in the Visforms code from Visforms version 5.1.0.
The above JavaScript code
- Hides the listbox listbox-age.
- Controls the listbox ‘listbox-age’ according to the date specified in field birth-date.